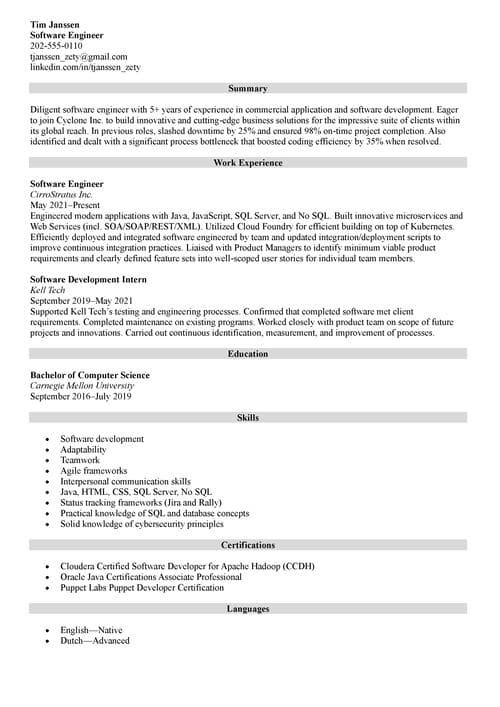
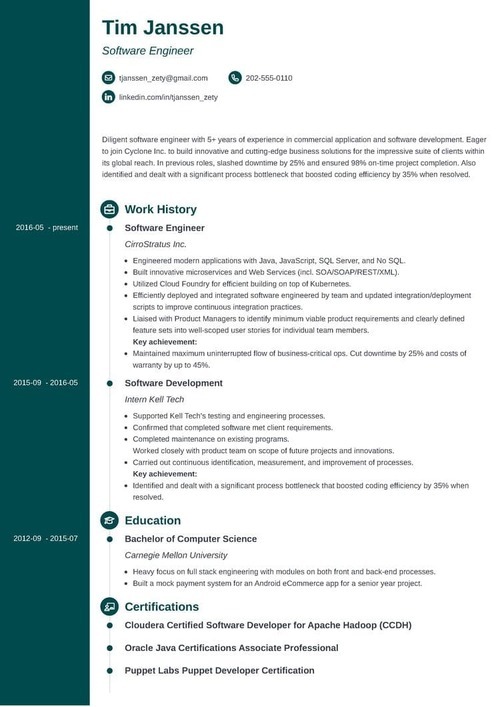
The JavaScript interview questions in this guide will set you up for success. Get the answers plus tips from CS hiring experts on what to focus on and how to stand above the rest.
These JavaScript interview questions and answers will set you up to land the job.
But—
You can’t just drill JavaScript coding interview questions and ace the interview. Who told us? CS hiring pros. Know what they said?
Most JS hiring managers won’t stop at asking JS interview questions. They want to know if you’re full-stack. They want employees who can think fast and deliver.
The good news?
It’s not hard to ace coding interviews like that.
This guide will show you:
Want to save time and have your resume ready in 5 minutes? Try our resume builder. It’s fast and easy to use. Plus, you’ll get ready-made content to add with one click. See 20+ resume templates and create your resume here.
Sample resume made with our builder—See more resume examples here.
Core advice:
Don’t just drill JavaScript interview questions. A coding interview is a job interview first. These guides can save your bacon:
1
Beware!
It’s not all coding!
You will face JavaScript coding interview questions in a JavaScript job interview.
But—
That’s not your only hurdle.
Here’s a tip from Sam Gavis-Hughson, founder of coding interview prep site Byte-by-Byte:
“Coding-specific questions are common at smaller companies, but big companies like Google and Facebook tend not to ask language-specific questions.”
Unicorns want well-rounded pros. They want full-stack, great, smart employees who know computer science back-to-front.
That said, you can’t walk into that JS jobs interview cold. Sharpen your coding chops with these advanced and basic JavaScript interview questions and answers:
Q: What will this innerHTML code output? Why?
<script>
function MessageA(){
document.getElementById('myMsg').innerHTML = 'Nice hat!';
}
function MessageB(){
document.getElementById('myMsg').innerHTML = 'Looks good on you though!';
}
</script>
<input type="button" onclick="MessageA()" value="You sir">
<input type="button" onclick="MessageB()" value="are a menace!">
<p id="myMsg"></p>
A: The output will be two buttons. One called “You sir” and the other “are a menace!” If we click the first, “Nice hat!” appears. If we click the second we see, “Looks good on you though!”
Q: What will this code output? Why?
var a = 2;
function outer() {
var a = 3;
function inner() {
a++;
var a = 4;
console.log(a);
}
inner();
}
outer();
A: The output is “4” because the inner function logs its own a variable.
Q: What could go wrong when using typeof demo === "object" to check if demo is an object? How can we avoid the problem?
A: Using typeof demo === "object" will tell us if demo is an object. But null is an object. So the following code will log true to the console:
var demo = null;
console.log(typeof demo === "object");
If we know this, we can check if demo is both an object and null:
console.log((demo !== null) && (typeof demo === "object"));
JavaScript doesn’t classify functions or arrays as objects. To include them in our search, we can amend the code like:
console.log((demo !== null) && ((typeof demo === "object") || (typeof demo === "function")) && (! $.isArray(demo)));
Or in JQuery we can say:
console.log((demo !== null) && (typeof demo === "object") && (! $.isArray(demo)));
Q: What’s the output of the code below in the console log? Why?
(function(){
var x = y = 7;
})();
console.log("x defined? " + (typeof x !== 'undefined'));
console.log("y defined? " + (typeof y !== 'undefined'));
A: The output is:
x defined? false
y defined? true
You might think typeof x and typeof y are undefined.
But that’s only if you think var x = y = 7 means:
var y = 7;
var x = y;
But actually it means:
y = 7;
var x = y;
In this case, y is a global variable. It’s in scope outside the function that created it. But we created x with var, so it’s a local variable. It’s undefined outside the function.
However, if we use strict mode, we’ll get a runtime error:
ReferenceError: y is not defined.
So—strict mode is our best bet for avoiding bugs in this case.
Q: Convert the string “8675309” to a number.
A: To convert a string to a number, we can use the Number() function:
var x = "8675309";
var y = Number(x);
Q: How would you get the value of an HTML textbox with JavaScript?
A: With the document.getElementById() method:
<html>
<head>
<script type="text/javascript">
function workWithTextBox() { var myBox = document.getElementById('MYBOX'); // perform action ... }
</script>
</head>
<body>
<input type="text" id="MYBOX">
</body>
</html>
Q: What will this code output and why:
console.log("87" === 87);
console.log("87" == 87);
console.log(false === 0);
console.log(false == 0);
A: The output will be:
false
true
false
true
In JavaScript, === means same value and type. But == tries to convert the values to the same type before comparing. In the first line, “87” is a string and 87 is a number, so it’s false. In the second, == converts “87” to 87 before comparing them.
The last two lines are more confusing. Of course false ===0 is false. But in the last line, == will convert both 0 and false into a false boolean.
For more on this confusing anomaly, see this SitePoint post.
Q: What’s the output from the code below and why?
var array1 = "sean".split('');
var array2 = array1.reverse();
var array3 = "brown".split('');
array2.push(array3);
console.log("First array length is " + array1.length + " and surname is " + array1.slice(-1));
console.log("Second array length is " + array2.length + " and surname is " + array2.slice(-1));
A: The output is:
First array length is 5 and surname is b,r,o,w,n
Second array length is 5 and surname is b,r,o,w,n
Because the two arrays are the same:
['n','a','e','s', ['b','r','o','w','n'] ]
That’s because:
Beware:
When we use the push() method to push array3 to array2, we add the entire array3 to the end of array2. We don’t concatenate the two arrays. (The concat() method does that.)
Q: In the following code, if the array is too large, we’ll get a stack overflow. How would you fix this without removing the recursive pattern?
var array1 = processBigArray();
var newArrayEntry = function() {
var entry = array1.pop();
if (entry) {
// do something to the current entry...
newArrayEntry();
}
};
A: We can avoid a stack overflow by changing the newArrayEntry function:
var array1 = processBigArray();
var newArrayEntry = function() {
var entry = array1.pop();
if (entry) {
// do something to the current entry...
setTimeout(newArrayEntry, 0);
}
};
That code eliminates the stack overflow because setTimeout(function, 0) clears the call stack before moving on to the next iteration.
Q: What will the code below output? Why?
<html>
<body>
<p id="x"></p>
<script>
try {
bar("Hi there!");
}
catch(error1) {
document.getElementById("x").innerHTML = error1.message;
}
</script>
</body>
</html>
A: The above code outputs: “bar is not defined” The catch block catches the error and displays it using the innerHTML() property.
Q: What is a hash table? Implement a simple one.
A: A hash table is a way to identify objects based on keys. We can implement a simple hash table like this:
var a = new Object();
a['first'] = 2;
a['second'] = 3;
a['third'] = 4;
// show values
for (var b in a) {
// filter keys
if (a.hasOwnProperty(b)) {
alert('key: ' + b + ', value: ' + a[b]);
}
}
Q: What is a “Singleton?” Implement a simple one.
A: A Singleton is when a class has one instance only, with a global point to access it. We can implement one with an immediate anonymous function:
var my_Single = (function () {
var iteration;
function createIteration() {
var obj = new Object("This is the iteration");
return obj;
}
return {
getInstance: function () {
if (!iteration) {
Iteration = createIteration();
}
return iteration;
}
};
})();
function run() {
var iteration1 = my_Single.getInstance();
var iteration2 = my_Single.getInstance();
alert("Same iteration? " + (iteration1 === iteration2));
}
Q: Write code to print all the numbers between 1 and 100. But in place of multiples of 3, print “Fizz” and for multiples of 5 print “Buzz.” When a number is a multiple of both, print, “FizzBuzz.” (Solution from CodeBurst.)
for (var i=1; i < 101; i++){
if (i % 15 == 0) console.log("FizzBuzz");
else if (i % 3 == 0) console.log("Fizz");
else if (i % 5 == 0) console.log("Buzz");
else console.log(i);
}
That FizzBuzz question is among the most popular JS interview questions employers ask. All it really does is test if you know the modulo operator %.
Pro Tip: Not sure if your JavaScript interview question code will work? Try this nifty online JavaScript tester. Plug in your code and watch it fly. Keep it open in a window for remote JavaScript interviews.
Ever heard of the STAR method for acing behavioral JavaScript basic interview questions? It’s hi-test. See our guide: STAR Method for Acing Behavioral Interview Questions (25+ Examples)
2
Prep for non-coding JavaScript interview questions or you’re cooked.
Why?
Well, picture this—
You drill 50 JS interview questions until you’ve got them cold.
But then the interviewer asks a bunch of general non-JavaScript coding interview questions.
What’s an abstract class? Is JS thread safe? Is it pass-by-value or pass-by-reference?
Uh, I don’t know. I can do it, but I can’t explain it.
Oh-oh.
Let these JavaScript basic interview questions get you exception-safe before the interview.
Q: What is innerHTML and what is it used for?
A: innerHTML is a JavaScript property used to set or get HTML content in an element node. For example, it can change the text in an HTML element from “Hello” to “World” based on some user action, like a button click.
Q: What is JavaScript typeof and how is it used?
A: We use the typeof operator to get the data type of an operand. Typeof tells if a function, variable, or object is an object, function, string, boolean, or undefined.
Q: How can you use JavaScript to set or get the contents of an HTML textbox?
A: With the HTML DOM input property getElementById().
Q: How can we find JavaScript string length?
A: With the string length property (syntax: var new_variable = old_variable.length;)
Q: How would you handle a list with JavaScript?
A: We handle lists in JavaScripts with arrays, set up with the syntax: var array_name = [entry1, entry2, ...];
Q: How many JavaScript array methods are there and which are the most useful?
A: There are 30 JavaScript array methods. Among the most useful are:
Pro Tip: Could you face “how does the internet work” as a JavaScript interview question? Yep. Bone up.
Q: What’s the difference between == and === in JavaScript?
A: Javascript has two equality tests: === and ==. The first one, === is strict equality. It checks if both the data and the type of are equal. If yes, JS returns true and if not, false. Meanwhile, == is loose equality. It tries to coerce the type before it checks. So, if we check 88=='88' the answer will be true, even though one is a number and the other a string.
Q: Is JavaScript thread safe?
A: JavaScript is single-threaded. Each execution thread finishes before the next starts, so JS has no thread safety issues.
Q: What is strict JavaScript and when and how should we use it?
A: JavaScript’s strict mode was introduced in ECMAScript5. It’s a way to opt out of “sloppy” JavaScript. It cleans up a lot of issues with non-strict code. Some browsers don’t support it so it must be used with rigorous testing. We invoke it by adding 'use strict'; to the start of a script.
Q: What’s the HTML DOM and how does JavaScript interact with it?
A: The HTML DOM is the Document Object Model. It’s the interface that lets JavaScript access and change the content and structure of a web page. JS uses different methods and events to dynamically change HTML, including document.getElementById() and innerHMTL.
Q: How do we print to console with JavaScript?
A: The JavaScript console object has methods that let us write directly to browser’s debug console, eg. console.log(). Debug console also lets us enter JS code directly into it, for quick testing.
Pro Tip: Have you got GitHub projects? Asking to talk about them is a favorite JavaScript interview question, so be ready. Review your favorites that fit the job.
Q: How would you change an array into a comma-separated list?
A: Use the array.join() method. To change the commas to a different separator, put the new separator between the parentheses.
Q: What’s the difference between && and || and ! in JavaScript? What are $ and !==?
A: The first three are logical operators meaning and, or, and not. The next, $, is a JavaScript identifier like any letter of the alphabet. It’s often used as an alias by JS libraries like JQuery. The last symbol, !==, means “not equal value or type.”
Q: How would you find a value in an array?
A: You could use the find() method to find the value or findIndex() to find the index of the element. Use Array.prototype.indexOf() or Array.prototype.includes() to find position or if an element exists.
Q: What is a memory leak in JavaScript? How do you detect one and how should you release memory?
A: A memory leak is a resource leak in JS. It happens when a program manages memory allocations incorrectly so unneeded memory isn’t properly released. We can detect them in Google Chrome by hitting F12, then using the memory tool’s Record Allocation Timeline feature to check for leaks. In JS, memory should be released by setting unused global variables to undefined or null so garbage collection can remove them. We can do this with an array called my_large_array with my_large_array=null.
Pro Tip: Does the company use React? Get ready for advanced JavaScript interview questions around how React uses higher order components or render props.
Q: What’s an abstract class? How is it different from an interface?
A: An abstract class is a class with an abstract method or methods. They aren’t standard in JavaScript, though we can implement them. Interfaces are programming structures that let the computer pass properties to an object (class).
Q: Does JavaScript support multiple inheritance?
A: No. Each object in JS has a single “prototype” object. However, we can add behaviors to classes with mixins.
Q: How would you find the intersection of 2 arrays of integers with JavaScript?
A: With Array.prototype.filter and Array.prototype.indexOf, like this: array1.filter(value => array2.includes(value))
Q: Is JavaScript pass-by-value or pass-by-reference?
A: JavaScript is always pass-by-value. Changing a variable’s value doesn’t change the underlying string or number. However, with objects the variable's value is a reference.
Q: Will console.log(0.1 + 0.2); produce 0.3? Why or why not?
A: No. It returns 0.30000000000000004 because JavaScript treats numbers with floating point precision.
Q: What are the six kinds of JavaScript exceptions?
A: They are:
Q: What is a closure?
A: A closure is a JavaScript feature where an inner function can access variables of the outer or “enclosing” function.
Q: How does “this” work?
A: In JavaScript, “this” disambiguates our code by pointing to the correct scope of the variable. It’s a kind of shortcut to a variable. It’s undefined until the function it’s defined in is invoked.
Need to brush up on more non-coding JS interview questions and answers? See the resources section at bottom.
Pro Tip: Are your JavaScript interview questions all about Vanilla JavaScript? Brush up on the basics at FreeCodeCamp.
Dig into the company before your interview. What frameworks do they use? What do they care most about? See our guide: 15 Best Informational Interview Questions to Ask (& Why They Matter)
When making a resume in our builder, drag & drop bullet points, skills, and auto-fill the boring stuff. Spell check? Check. Start building a professional resume template here for free.
When you’re done, Zety’s resume builder will score your resume and tell you exactly how to make it better.
3
Heads up!
They might not ask any JavaScript coding interview questions.
At least, not like the ones in job search articles.
Instead, they might give you a few programming assignments.
These next few advanced JavaScript interview questions for experienced applicants will validate your JS skills.
Design an algorithm that normalizes a string containing a URL. Our URL list has become corrupted so the URLs have double dots (..) and triple slashes (///). Use JavaScript to remove all extra characters from the string.
Create an algorithm that multiplies two very large numbers that wouldn’t fit into ordinary data types and are given as a string.
Design an algorithm that uses JavaScript to shuffle a pack of cards.
Using JavaScript, write an algorithm that finds and displays a user’s ZIP code.
Still worried you can’t ace basic JavaScript interview questions? Don’t sweat it much. Seasoned developers remind us that ignorance of one programming language usually isn’t a deal-breaker.
Big fish like Amazon look for “fungible” developers. That means they want engineers who can work across the stack, are smart, and can quickly pick up on new things.
Fundamental computer science concepts matter most. Brush up on data structures and object-oriented programming concepts like inheritance, polymorphism, abstraction, encapsulation, and recursion.
Pro Tip: Don’t know the answer to a JavaScript coding interview question? Think out loud. “I’d probably try this and if that failed I’d do some Googling and testing. It’d take me about twenty minutes to get it right.”
What makes you special? Yep, that’s a legit JavaScript interview question. See our guide: How to Answer What Makes You Unique—Samples & Interview Guide
4
They’ll probably give some math and logic puzzles with their JS interview questions.
Why?
To see if you can make a reasoned estimate based on logic and available data.
In other words, are you smart? Or do you just know how to code?
Use these math/logic JavaScript interview questions and answers to prep for inevitable curve balls.
Q: How many gas stations are in the United States?
Why they ask: To see if you can make good estimates based on available data. You might see variations on this JavaScript interview question.
A: Well, I grew up in a town of about 20,000, and I’d say there were about 20 gas stations. That means about one station for every 1,000 people. So if that holds true for the rest of the country, and the US population is 330 million, we’d have about 165,000 gas stations. (In fact there are 168,000.)
Q: You have 10 bottles with 100 pills each. Nine of every 10 bottles have pills that weigh 1 gram, but one in 10 has pills that weigh 1.1 grams. How could you find the heavy bottles if you could use a scale only once?
Why they ask: To test your math and problem-solving skills.
A: Take one pill from bottle #1, two from #2, and so on for a total of 55 pills. If they were all light, they’d weigh 55 grams. But if the heavy pills are in bottle #1, you’ll have 55.1 grams. If in bottle #2, 55.2 grams, and so on.
Q: How would you find a specific book in a large library with no cataloguing system?
Why they ask: To see if you can make good decisions in the face of open-ended problems.
A: Without a cataloguing system, the only guaranteed way to find the right book is an exhaustive search. Assuming time is limited, we should look for storage patterns. For instance, we could check the first three books of every 15th row. If we still don’t see a pattern, we could vary our granularity and check every 5th or 30th row. We could speed the process up through parallelization (having friends search different parts of the library.
Q: Which way should a car key turn to unlock the door?
Why they ask: To see if you’ll waste time on insignificant problems.
A: The “right” answer is that it should turn right, since there are more right-handed people. But it’s arbitrary, so you shouldn’t waste a lot of time on it.
Q: You have to measure 4 gallons of water, but you only have a 5-gallon jar and a 3-gallon jar. How can you do it?
Why they ask: To test your math and logic skills.
A: Fill the big jar. Fill the small jar from it, then empty the small jar. Pour the two gallons from the big jar into the small jar. Fill the big jar again. Use it to fill the small jar to the brim. Now there are four gallons of water in the big jar.
Want more math and logic puzzles to get ready for JS interview questions? CrazyforCode has a great list.
Pro Tip: Vying for a job at CloudGuru? They use JavaScript (and particlularly Node.JS) heavily in their serverless web applications and courses.
“Tell me about yourself.” Ugh. The worst of all JavaScript interview questions. See our guide: Tell Me About Yourself: Interview Question & How to Answer [Example]
5
Why study general computer science concepts along with JS interview questions?
To quote Yoda:
Not ready are you.
“Full stack” means you’re not just limited to JavaScript. You didn’t just take some W3Schools lessons and fill out the application.
They want to know you’re three-dimensional.
Use these basic JavaScript interview questions and answers to prove it.
Q: What does “exception safe” mean?
A: Exception safe code follows guidelines to handle exceptions so the software explains its bugs and errors.
Q: In an object-oriented language, what’s the difference between “HAS-A” and “IS-A?” In other words, define inheritance vs ownership.
A: An IS-A relationship is based on inheritance as in “X is a Y kind of thing.” A HAS-A relationship is based on references, like “fish have fins” or “cars have wheels.”
Q: What’s a “priority queue?”
A: Priority Queue is a queue where each item has a priority, a high-priority element gets dequeued before low-priority elements, and elements with the same priority are served depending on their order within the queue.
Q: What does “dequeue” mean?
A: To remove or process an item from a queue.
Q: What is Big O and why is it important?
A: Big O is a notation for an algorithm’s complexity or performance. O(1) would be an algorithm that uses the same amount of processing resources no matter how much data gets thrown at it. O(N) algorithms take more time to crunch bigger data sets, in a linear relationship. O(N2) is an algorithm where the processing power needed is in direct proportion to the square of the data set size. O(2N) means growth doubles with every addition to the data set.
Pro Tip: Get ready for a few Node JavaScript interview questions. Brush up on the event loop, graphQL, and some general HTTP questions.
Q: What is polymorphism and why is it important?
A: Polymorphism is a tenet of Object Oriented Programming (OOP). It’s where we design objects with shared behaviors we can override in special cases. Polymorphism uses inheritance. It’s a core concept in OOP because it vastly simplifies programming
Q: What are the different OOPS principles?
A: They are:
Q: What is inheritance?
A: Inheritance is when a parent class passes a property or properties to a child class.
These questions require you to prove advanced IT skills. Are you sure you have them all? Check here:Technical Skills List: What Employers Want.
6
They’re not the only ones who should ask JavaScript interview questions.
Ask the hiring manager some questions too. You need to show you know your stuff. But you also need a snapshot of what it’s like to work there. Try:
If they’re happy to talk about this stuff, it’s probably a good company. If they get agitated, they’ve got monsters in the closet, so beware.
A lot of companies these days use JS frameworks. Their JS interview questions will be particular to whatever framework they use (eg Angular).
So—
Before the interview, find out if they use a JS framework and study up on it.
Want to drill more JavaScript coding interview questions? Try the $13 Udemy course in the next section. It’s our fav.
Pro Tip: Confidence is key when answering advanced JavaScript interview questions. Don’t be terrified of making a mistake. We all do it and good employers understand.
Need more questions to ask an interviewer in a JavaScript jobs interview? See our guide: 65+ Best Questions to Ask an Interviewer & Land Top Jobs
7
Still feeling wobbly? Not ready to answer all the JavaScript interview questions you’ll face?
If your JS still has chinks, take heart.
Any of the excellent resources below will set you up in Hulkbuster armor.
Pro Tip: How’s your CSS? You’d better believe they can quiz you on it amid the other JavaScript interview questions.
What’s your greatest weakness? Kryptonite and inane JavaScript coding interview questions? See our guide: "What Is Your Greatest Weakness?" Best Answers (6 Proven Examples)
Plus, a great cover letter that matches your resume will give you an advantage over other candidates. You can write it in our cover letter builder here. Here's what it may look like:
See more cover letter templates and start writing.
Here’s how to prepare for different JavaScript Interview Questions and ace your answers:
Still worried about the hairy JavaScript interview questions they might throw at you? Got JS interview questions we didn’t think of? Give us a shout in the comments. We’d be glad to chat!
Modern resume design for the modern day resume. See creative samples and follow our guide to make the best modern resume that will land you the job.
A step-by-step guide to writing an IT resume. 20+ actionable examples and tips from experts. Use our sample resumes for IT jobs, complete with an IT resume-writing tutorial, job description advice, skills to include, and more. Get started on your information technology career now!
Learn what kind of C# questions to expect at a technical interview, why they are asked, and how to prepare. We reached out to IT pros to help you out!